Simple JavaScript Games For Beginners
In order to learn JavaScript programming, you need to have a basic understanding of how the language works. JavaScript is a “loosely typed” language, which means that you don’t need to worry about the data type of a variable (ie. whether it’s a number, string, or boolean). This makes the language more forgiving and easier to learn for beginners. This blog post will introduce you to some simple javascript games.
One of the best ways to learn a programming language is by writing code to create simple games. Games are fun and engaging, and they can help you learn the basics of JavaScript programming. In this article, we’ll show you how to code a basic JavaScript game.
How To Create The Ancient Game Tic-Tac-Toe Using Javascript
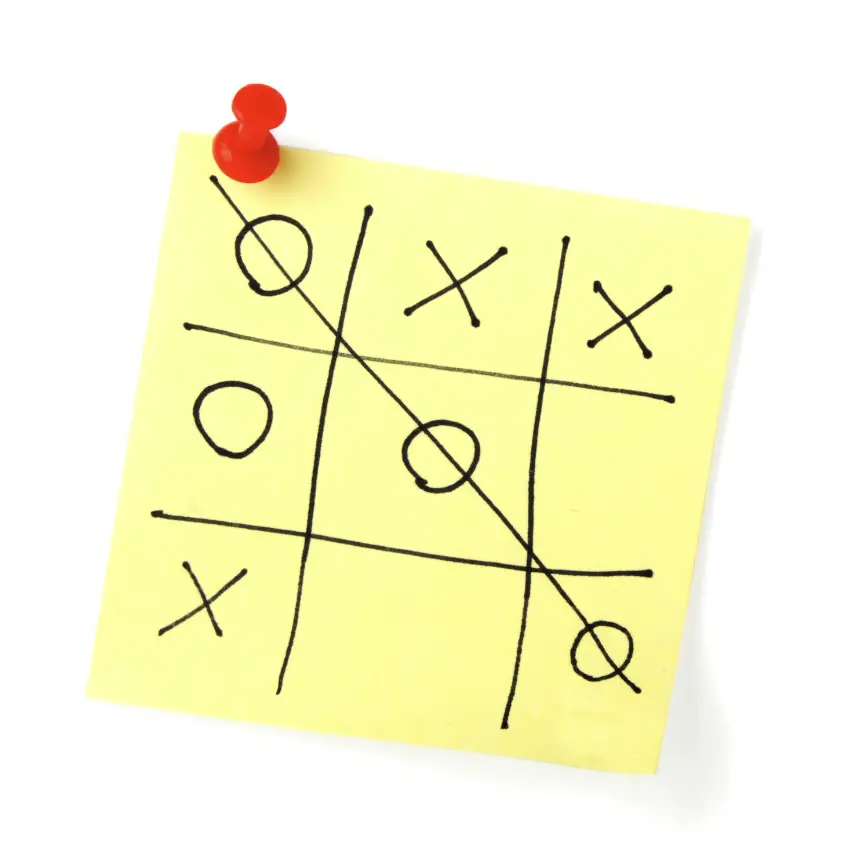
As it turns out, Tic-Tac-Toe can be traced back to Egypt, circa 1300 BC. Clearly, it has withstood the test of time! In order to create a tic tac toe game today using JavaScript, you will need to write code to create the following elements:
- A title screen that displays the game title
- A grid that displays the game board
- Buttons that allow the player to input moves
- A message area that displays the player’s score and status
The game logic for tic tac toe is simple. The player who gets three of their symbols in a row (horizontal, vertical, or diagonal) wins the game. If all nine spaces on the grid are filled and no player has won, then the game is a tie.
To write code for the tic tac toe game, you will need to use the following JavaScript concepts:
- Variables: to store data such as the player’s name, score, and moves
- Functions: to modularize your code and make it easier to read
- Control flow: to manage the sequence of events in the game
- User input: to allow the player to make moves
When you have finished writing your code, you can test the game by playing it yourself. You can also share it with your friends to see who can get the best score!
Using JavaScript To Code Minesweeper Game
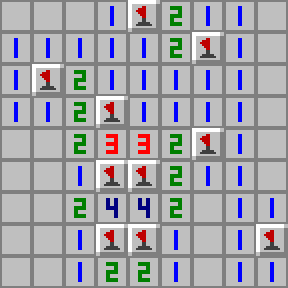
Minesweeper is a classic game that can be easily recreated using JavaScript. The goal of the game is to uncover all of the mines on the board without detonating any of them.
To create a minesweeper game, you will first need to create a new HTML document and include a <script> tag. Within the <script> tag, you will need to write a function that will handle the user’s input. This function will take in the x and y coordinates of the user’s click and determine whether or not there is a mine at that location.
If there is a mine at the location, the function should display an alert message telling the user they have hit a mine. If there is not a mine at the location, the function should update the HTML document to reveal the number of mines that are adjacent to the revealed square.
Once all of the mines have been revealed, the game is over and the user has won!
How About Hangman With JavaScript?
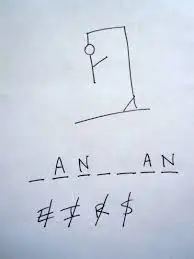
The game of Hangman is a simple guessing game. The player guesses a letter, and the computer responds with a series of blanks, each representing a letter in the word. If the player guesses a letter that appears in the word, the computer fills in the blank with that letter. If the player guesses a letter that does not appear in the word, the computer adds a body part to the stick figure. The player wins if they can guess the word before the stick figure is complete.
In order to make hangman with JavaScript, you will need:
- An html file
- A javascript file
- A CSS file
- An image for the hangman game (optional)
The key to Hangman in JavaScript are the code strings and proper management of user input. A dictionary library is very useful here.
JavaScript Simple Games: Reaction And Memory
The reaction game works by asking the player to choose one of two possible responses to a question. If the player chooses the correct response, they earn points and the game continues. If the player chooses the incorrect response, they lose points and the game continues.
The memory game works by presenting the player with a series of images. The player must then remember the order in which the images were presented and reproduce that order. If the player correctly remembers the order, they earn points and the game continues. If the player cannot correctly remember the order, they lose points and the game continues.
Both of these games can be played indefinitely, with the player’s score being tracked throughout. These games are simple but provide a good challenge for beginner JavaScript programmers.
Have fun!