Java – Arrays
Multiple values can be stored in a single variable using arrays. In Java, an array’s items must all be of the same datatype.
Create an Array
It begins by declaring the array’s datatype, which is encased in square brackets [], and then the array’s name.
Initialize an Array
A comma can be used to separate the items of Java Arrays, and curly brackets can be used to enclose them. Please keep in mind that declaring an array does not allocate memory for it. An array must be initialised before it may be allocated memory. If the array was not initialised when it was declared, it must be initialised with the new keyword, followed by the data type and array size wrapped in square brackets []. For numerous declaration and initialization procedures, check the syntax below.
Syntax
//both declaration methods are valid in Java int[] MyArray; int MyArray[]; //declaration and initialization of //an integer array separately int[] MyArray; MyArray = new int[5]; //size of array is 5 MyArray[0] = 10; //first element is 10. //declaration and initialization of an string //array in a single line String[] MyArray = {"MON", "TUE", "WED"}; //Another method of declaration and initialization //of an string array in a single line String[] MyArray = new String[5];
Access element of an Array
The index number of an array element can be used to access it. In Java, an array’s index number begins with 0 as illustrated in the diagram below:
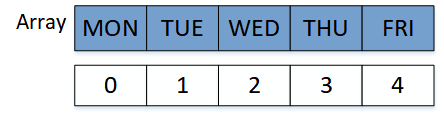
The following example shows how to use an array’s index number to retrieve its elements.
public class MyClass { public static void main(String[] args) { //creating an array with 5 elements String[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; //accessing the element with index number 4 System.out.println(weekday[4]); } }
The output of the above code will be:
FRI
Modify elements of an Array
Any element of an Array can be changed by assigning a new value to its index number.
public class MyClass { public static void main(String[] args) { String[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; weekday[0] = "MON_new"; weekday[3] = "THU_new"; System.out.println(weekday[0]); System.out.println(weekday[3]); } }
The output of the above code will be:
MON_new THU_new
Number of elements in Array
The length property of an array may be used to estimate the number of elements in the array.
public class MyClass { public static void main(String[] args) { String[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; System.out.println(weekday.length); } }
The output of the above code will be:
5
Loop over an Array
We may access each element of an Array using a for loop or a while loop. We may also use the for-each loop, which is only available for looping through the items of an array.
For Loop over an Array
The for loop is used in the example below to access all items of the provided array.
public class MyClass { public static void main(String[] args) { String[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; for(int i = 0; i < weekday.length; i++) { System.out.println(weekday[i]); } } }
The output of the above code will be:
MON TUE WED THU FRI
While Loop over an Array
Similarly, the while loop may be used to access array items.
public class MyClass { public static void main(String[] args) { int[] numbers = {10, 20, 30, 40, 50}; int i = 0; while(i < numbers.length) { System.out.println(numbers[i]); i++; } } }
The output of the above code will be:
10 20 30 40 50
For-each over an Array
Syntax
for(datatype i : MyArray){ statements; }
The for-each loop is used in the example below to access all items of the provided array.
public class MyClass { public static void main(String[] args) { int[] numbers = {10, 20, 30, 40, 50}; for ( int i : numbers) { System.out.println(i); } } }
The output of the above code will be:
10 20 30 40 50
Multi-dimensional Array
Arrays of arrays can be considered multi-dimensional arrays. You can define arrays of any size in Java. To access items of a multi-dimensional array, use a For-loop or While-loop.
Syntax
//2-dimension array int[][] MyArray; //3-dimension array int[][][] MyArray; //2-dimensional array int[][] MyArray = {{10,20,30},{100,200}};
public class MyClass { public static void main(String[] args) { int[][] numbers = {{10, 20, 30}, {40, 50, 60}}; for(int i = 0; i < 2; i++) { for(int j = 0; j < 3; j++) { System.out.println(numbers[i][j]); } } } }
The output of the above code will be:
10 20 30 40 50 60