Python – For Loop
The For loop
In Python, the for loop iterates over a sequence, executing a series of instructions for each element in the sequence. Any data structure, such as a list, tuple, set, string, dictionary, and range iterables, can be used to create a sequence.
Syntax
for iterating_var in sequence: statements
Flow Diagram:
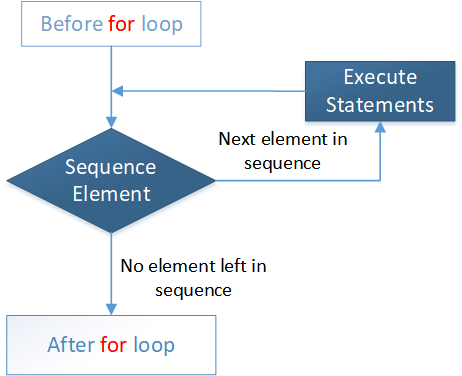
For loop over a range:
The Python – For Loop is used in the example below to loop over a set of values.
print("#Result 1:") for i in range(4): print(i, i * 2) print("\n#Result 2:") for j in range(2, 6): print(j, j ** 2) print("\n#Result 3:") for k in range(10, 2, -2): print(k)
The output of the above code will be:
#Result 1: 0 0 1 2 2 4 3 6 #Result 2: 2 4 3 9 4 16 5 25 #Result 3: 10 8 6 4
For loop over List
The for loop is used to access the elements of a list in the example below.
colors = ['Red', 'Blue', 'Green'] for x in colors: print(x)
The output of the above code will be:
Red Blue Green
For loop over Tuple
The for loop is used to access the elements of a tuple in the example below.
days = ('Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday') for day in days: print(day)
The output of the above code will be:
Monday Tuesday Wednesday Thursday Friday Saturday Sunday
For loop over Set
numbers = {100, 200, 300, 400, 500} for i in numbers: print(i)
The output of the above code will be:
400 100 500 200 300
For loop over String
word = 'python' for letter in word: print(letter)
The output of the above code will be:
p y t h o n
For loop over Dictionary
A for loop may be used to access key-value pairs in a dictionary in the same way.
dict = { 'year': '2000', 'month': 'March', 'date': 15 } print("#Result 1:") for day in dict: print(day) print("\n#Result 2:") for day, value in dict.items(): print(day,value)
The output of the above code will be:
#Result 1: year month date #Result 2: year 2000 month March date 15