How to check number is even or odd using python code
In this tutorial, we are going to show How to check number is even or odd using python. We have added the video tutorial and the source code of the program.
Video Tutorial: Check number is even or odd python code
Algorithm
There are a few different ways to check if a number is even or odd. One way is to use the modulus operator, %, to see if the number is divisible by 2. For example, the number 6 is even because it is divisible by 2, but the number 7 is odd because it is not divisible by 2. Another way to check if a number is even or odd is to use the bitwise operators, & and |. The number 6 is even because it has a 0 in the least significant bit, and the number 7 is odd because it has a 1 in the least significant bit.
Python’s even/odd algorithm is a very simple way of determining whether a number is even or odd. The algorithm takes in a number and determines whether the number is evenly divisible by two. If it is, then the number is even. If it is not evenly divisible by two, then the number is odd.
The code for the Python even/odd algorithm is very simple. It is a one-liner and can be easily implemented into any Python program.
def is_even(n): return n % 2 == 0
Source Code
# Python program to check if number is even or odd number = int(input("Enter a number: ")) if (number % 2) == 0: print(number,"is Even number") else: print(number,"is Odd number")
Output
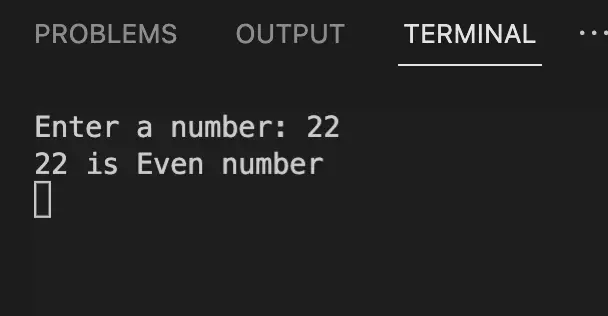